表单
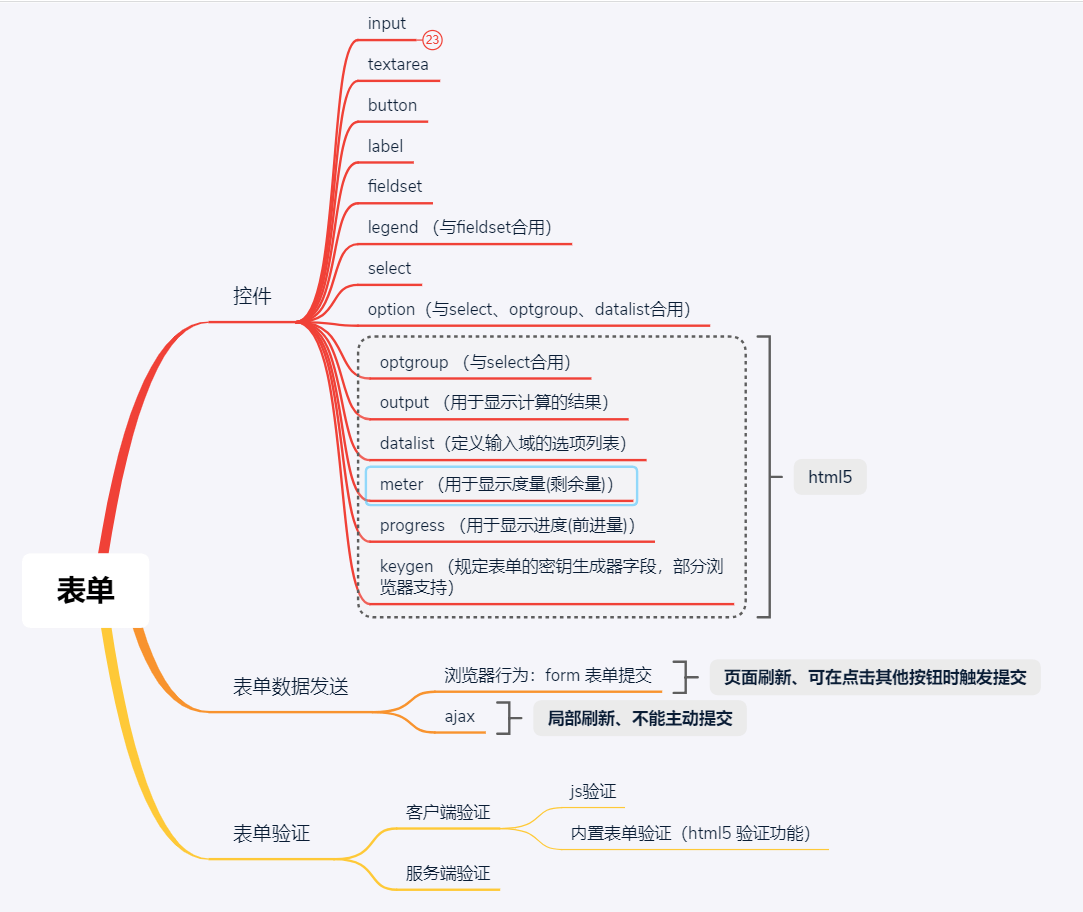
点击图片查看完整分类
有关文档:
MDN - Web forms
MDN - HTML表单指南
MDN - HTML elements reference (可以查看所有html元素)
Tips: 中文版翻译没跟上英文版,建议直接看英文版。
# 表单数据发送
# 浏览器行为
通过设置<form>
有关属性 action、method 等,触发浏览器行为,从而发送数据
<form action="/api/user/add" method="post" enctype="text/plain">
<label for="say">What greeting do you want to say?</label>
<input name="say" id="say" value="Hi">
<button>Send my greetings</button>
</form>
2
3
4
5
# FormData
通过FormData() 构造函数创建一个新的FormData对象,从而获得表单值。
<form id="myForm" name="myForm">
<label for="username">Enter name:</label>
<input type="text" id="username" name="username">
<input type="button" id="btn" value="提交">
</form>
<script>
document.getElementById('btn').onclick = function () {
var myForm = document.getElementById('myForm');
formData = new FormData(myForm); // 此时formData包含了myForm的表单值
var xhr = new XMLHttpRequest();
xhr.open('POST', '/api/form');
xhr.responseType = 'json';
// xhr.setRequestHeader('Content-Type', 'multipart/form-data'); 不需要设置
xhr.send(formData);
xhr.onload = function () {
console.log(this.response);
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
注意
FormData 接口提供了一种表示表单数据的键值对的构造方式,经过它的数据可以使用
XMLHttpRequest.send()
方法送出。所有输入元素都需要带有
name
属性,否则无法访问到值。使用FormData收集表单数据提交给服务器,一定要选择POST方式,且不需要设置
Content-Type
,浏览器会自动识别并添加Content-Type: multipart/form-data
。
# enctype 和 Content-Type
enctype: <form>
的一个属性,该属性规定在发送到服务器之前应该如何对表单数据进行编码,可取值有:
- application/x-www-form-urlencoded:在发送前对所有字符进行编码(默认)
- multipart/form-data:不对字符编码。当使用文件上传控件表单时,该值是必须的。
- text/plain:将空格转换为 "+" 符号,但不编码特殊字符
Content-Type(MediaType),即是Internet Media Type,互联网媒体类型,也叫做MIME类型。 在HTTP协议消息头中,使用Content-Type来表示请求和响应中的媒体类型信息。它用来告诉服务端如何处理请求的数据, 以及告诉客户端(一般是浏览器)如何解析响应的数据,比如显示图片,解析并展示html等等。 常见的值有:
- application/x-www-form-urlencoded
- multipart/form-data:表单数据都保存在http的正文部分,各个表单项之间用boundary分开。多用于文件上传
- application/json:消息主体是序列化的json字符串
- ...
联系:在文件上传时,需要设置为multipart/form-data。如果使用了FormData(),则不需要手动设置,浏览器会自动识别并添加Content-Type: multipart/form-data
# URLSearchParams
URLSearchParams 接口定义了一些实用的方法来处理 URL 的查询字符串。
var paramsString = "q=URLUtils.searchParams&topic=api"
var searchParams = new URLSearchParams(paramsString);
for (let p of searchParams) {
console.log(p);
}
searchParams.has("topic") === true; // true
...
2
3
4
5
6
7
8
9
10
有关文档
MDN - FormData
MDN - URLSearchParams