数据类型
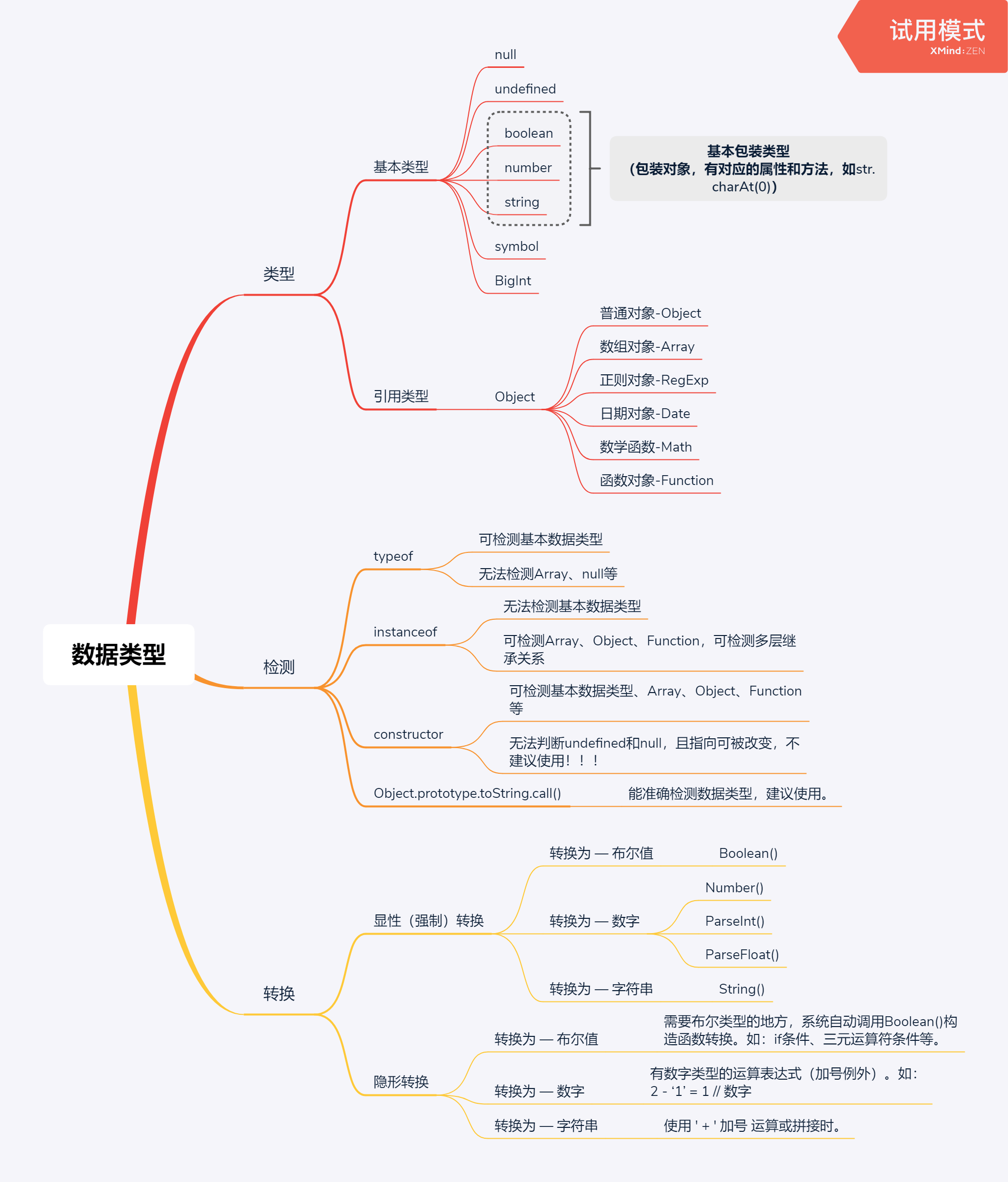
# 数据类型检测
# typeof
可检测基本数据类型和function,无法检测引用数据类型
var arr = [
null, // object
undefined, // undefined
true, // boolean
12, // number
'haha', // string
Symbol(), // symbol
20n, // bigint
function(){}, // function
{}, // object
[], // object
]
for (let i = 0; i < arr.length; i++) {
console.log(typeof arr[i])
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# instanceof
无法检测基本数据类型,可检测function 、引用类型和继承关系
var arr = [
// { 'value': null, 'type': null}, ----> error
// { 'value': undefined, 'type': undefined}, ----> error
{ 'value': true, 'type': Boolean}, // false
{ 'value': 12, 'type': Number}, // false
{ 'value': 'haha', 'type': String}, // false
{ 'value': Symbol(), 'type': Symbol}, // false
{ 'value': 20n, 'type': BigInt}, // false
{ 'value': function(){}, 'type': Function}, // true
{ 'value': {}, 'type': Object}, // true
{ 'value': [], 'type': Array}, // true
]
for (let i = 0; i < arr.length; i++) {
console.log(arr[i].value instanceof arr[i].type)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
instanceof 除了可以检测类型外,还可以在继承关系的检测中使用
function Aoo(){}
function Foo(){}
Foo.prototype = new Aoo(); // JavaScript 原型继承
var foo = new Foo();
console.log(foo instanceof Foo) // true
console.log(foo instanceof Aoo) // true
1
2
3
4
5
6
7
2
3
4
5
6
7
# constructor
提示:虽可检测,但 prototype 可被改写,constructor 会改变,不建议使用该方法判断。
var arr = [
// { 'value': null, 'type': Null}, ----> error
// { 'value': undefined, 'type': Undefined}, ----> error
{ 'value': true, 'type': Boolean}, // true
{ 'value': 12, 'type': Number}, // true
{ 'value': 'haha', 'type': String}, // true
{ 'value': Symbol(), 'type': Symbol}, // true
{ 'value': 20n, 'type': BigInt}, // true
{ 'value': function(){}, 'type': Function}, // true
{ 'value': {}, 'type': Object}, // true
{ 'value': [], 'type': Array}, // true
]
for (let i = 0; i < arr.length; i++) {
console.log(arr[i].value.constructor == arr[i].type)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# Object.prototype.toString.call()
一般类型都能检测,建议使用。
var arr = [
null, // [object Null]
undefined, // [object Undefined]
true, // [object Boolean]
12, // [object Number]
'haha', // [object String]
Symbol(), // [object Symbol]
20n, // [object BigInt]
function(){},// [object Function]
{}, // [object Object]
[], // [object Array]
new Date(), // [object Date]
new RegExp(),// [object RegExp]
new Error(), // [object Error]
]
for (let i = 0; i < arr.length; i++) {
console.log(Object.prototype.toString.call(arr[i]))
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
# 数据类型转换
经典面试题
1 + '1'
true + 0
{}+[]
4 + {}
4 + [1]
'a' + + 'b'
console.log ( [] == 0 )
console.log ( ! [] == 0 )
console.log ( [] == ! [] )
console.log ( [] == [] )
console.log({} == !{})
console.log({} == {})
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12